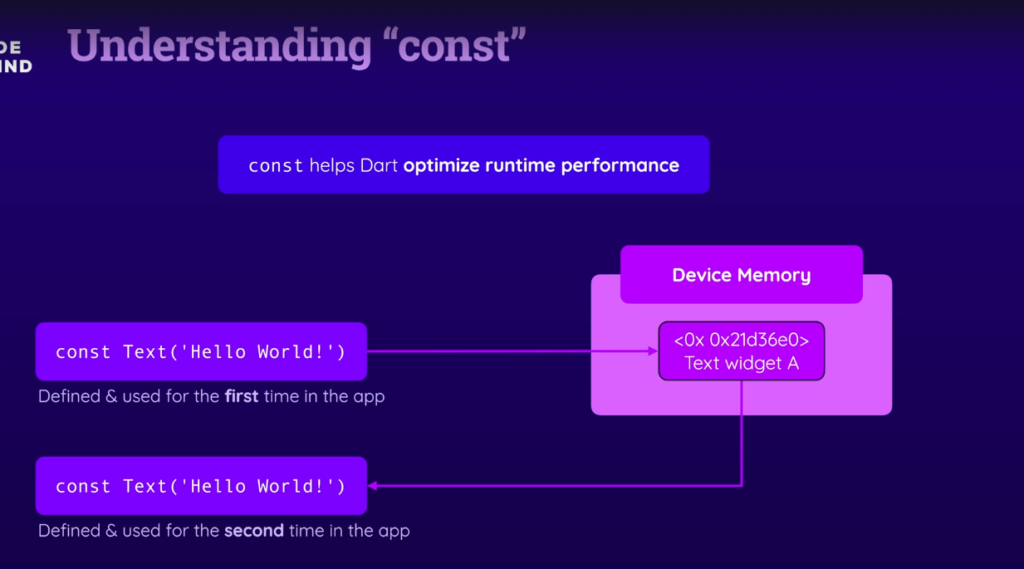
Understanding Const
The const keyword helps Dart in optimizing the runtime performance of the app, as the widget that is defined as const will be stored in the device memory. So, whenever the same widget is required again in the widget tree/app then in that case instead of re-rendering the widget and getting its values, making object allocation and recalculating the values leading to a smoother performance.
Data types
Dart is a type safe language and it requires that only the required types by a function are passed to the function or passed from the function, every variable, constant or widget is type of Object and it can have multiple possible types . For ex :
int a = 434;
here “a” has the type of num, int as well as Object type.
Some of the core types that are supported in Flutter because of Dart are :
There are also a few other built-in types along with these core types:
- Records ( (value1, value2))
- List (Also known as Arrays)
- Sets
- Maps
- Runes
- Symbol
- null
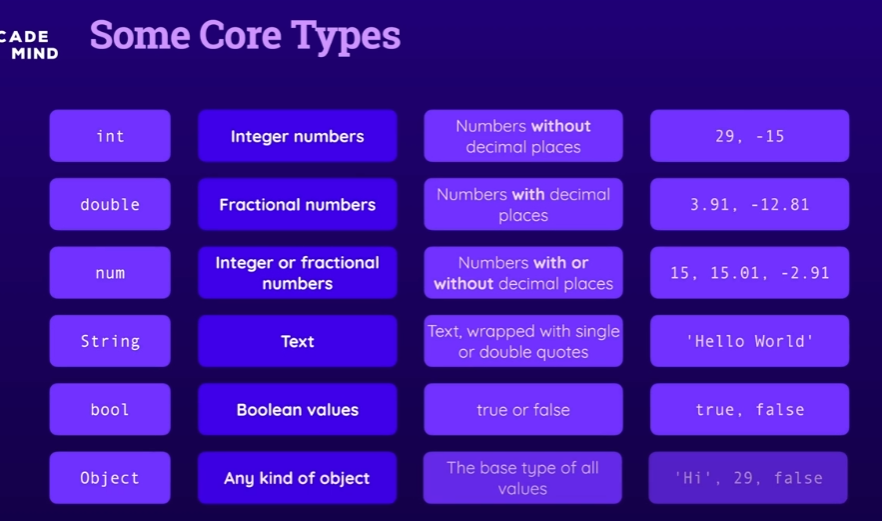
void as a special data type in flutter
In Flutter (and Dart, the language Flutter is built on), void
is not considered a data type in the same way that int
, String
, or bool
are. Instead, void
is a special type that signifies the absence of a return value. When you see void
in a function definition, it means that the function does not return any value.
Here’s an example to illustrate this:
void myFunction() {
print('This function does not return a value.');
}
In this example, myFunction
is defined with a return type of void
, indicating that it does not return anything when called. You might compare this to void
in other programming languages like C, C++, or Java.
Key Points About void
in Dart:
1.No Return Value: Functions with a void
return type do not return a value. If you try to return a value from such a function, you will get a compile-time error.
2.Usage in Callbacks: Often used in callbacks where no return value is needed.
3.Compatibility with Future<void>
: In asynchronous programming with Dart, you can also have functions that return Future<void>
, which means they perform asynchronous operations but do not return a value.
Example with Future<void>:
Future<void> asyncFunction() async {
await Future.delayed(Duration(seconds: 2));
print('This is an asynchronous function that does not return a value.');
}
In this example, asyncFunction
performs an asynchronous operation (waiting for 2 seconds) and then prints a message, but it does not return a value.
Overall, void
is a way to indicate that a function performs some operations but does not provide any result that can be used by the caller.
The “const” keyword helps Dart in optimizing the runtime performance of the app, as the widget that is defined as const will be stored in the device memory and whenever the same widget is required again in the widget tree/ app then in that instead of re-rendering the widget and getting its values , making object allocation and recalculating the values leading to a smoother performance.